Loading images
Make your App more interesting with pictures
Making an app with text only is not exactly exciting. Now we're going to add some graphics to it - still not too exciting, but a step in the right direction.
The most common scenario, especially if you're making a game, is one where you load a bunch of resources first, and then initialize the app when the resources are ready. We can do this easily with WADE, by adding a load function to our App, and loading resources in there like this:
App = function() { this.load = function() { wade.loadImage('clubs.png'); }; }
Note that for this to work you'll need a file called clubs.png (download it here) in the same folder as everything else. You can put it in a subfolder too (I think that would be a good idea), and you can then load it using a relative path. So, for example, if you've saved your file in a subfolder called data, you can load it with:
wade.loadImage('data/clubs.png');
You can also use web paths for your resources, like this:
wade.loadImage('/assets/tutorial/clubs.png');
However be aware that many browsers implement all sorts of security-related restrictions for resources that come from different domains. If you want to use a remote resource and just display it on the screen it's fine, but if you wanted to inspect it to see whether any pixel is transparent, for example, your browser wouldn't let you do that, because of something called Same Origin Policy. In general, it's good practice to just place all your resources in a subfolder of your project, to avoid these restrictions altogether.
WADE supports the most common image formats - in fact, it supports all the image formats that your browser supports. Using .png, .jpg and .gif is usually a good idea, as they are supported everywhere. Other formats may or may not be supported depending on the browser that the user is running.
So we've loaded an image. Now it's time to display it on the screen. We can do this at the end of our init function, by doing the same thing that we've done with our "hello world" text: first, we create a Sprite object (not a TextSprite this time), then a SceneObject that contains it, and then we add the SceneObject to the scene:
var clubsSprite = new Sprite('data/clubs.png'); var clubsObject = new SceneObject(clubsSprite); wade.addSceneObject(clubsObject);
Now we should have something like this:
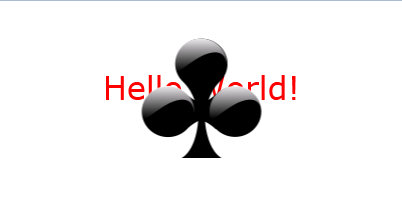
This is how it works: when WADE is initialized it asks our app to load any resources that it may need, and it does so by calling its load function. In this function, we can load as many resources (images, audio files, scripts, etc.) as we need. When they're all finished loading, WADE calls our init function.
WADE handles all the error-checking for us, so if there were network errors and it failed to load an image, it would automatically try again a few times before giving up. But we don't have to worry about that: when our init function is executed, we can be sure that our resources have been loaded.
WADE also supports asynchronous loading of resources in the background (so you can run your app while loading more resources), but that's a topic for a future tutorial.
That's pretty much all you need to know about loading images for now. You can do the same with scripts (.js files), JSON data (.json files), audio, etc. using the appropriate functions (wade.loadScript, wade.loadJson, wade.loadAudio).
To conclude this first chapter of the WADE tutorials, we'll just make the clubs sprite a bit smaller and we'll move it a bit to the left, so it doesn't overlap our "Hello World" text.
var clubsSprite = new Sprite('data/clubs.png'); clubsSprite.setSize(64, 64); var clubsObject = new SceneObject(clubsSprite); wade.addSceneObject(clubsObject); clubsObject.setPosition(-100, 0);
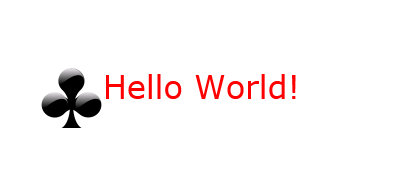
Try it right here
As an exercise, you could try to create a second sprite (still using the clubs.png image) and positioning it to the right of the "Hello World" text.